ja_webutils - a Python package for building HTML docments
Overview
ja_webutils is a collection of classes that are used to programmatically create html pages. These classes can be considered medium level abstractions.
The overriding design philosophy is to record in a class, method or attribute anything we had to search the Web to learn how to do. This doesn’t preclude including raw html directives.
The canonical hello application could look like:
import webbrowser
from pathlib import Path
from ja_webutils.Page import Page
page = Page()
page.title = 'Hello'
page.add('Hello world')
html = page.get_html()
ofile = Path('/tmp/ja_webutils_example_1.html')
with ofile.open('w') as ofp:
print(html, file=ofp)
webbrowser.open_new_tab(f'file://{ofile.absolute()}')
The resulting html file looks like:
<!DOCTYPE html>
<html dir="ltr" lang="en">
<head>
<meta charset="UTF-8">
<title>Hello</title>
</head>
<body >
Hello world<br><br>
<p class="footer" > Page generated in 0.00s on 2023-09-06 16:31:27 UTC</p>
</body>
</html>
Which produces:
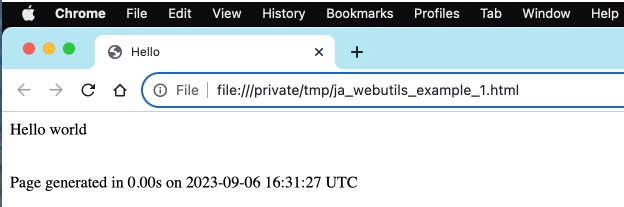
Fig. 1 Browser (Chrome) rendition of the above example
The overall organization of the package:
The Page
class is a container that will produce complete HTML with a <head> and <body> section.
It allows style and javascript to be added as strings or loaded from content delivery sites,
including your own. This allows our PageTable
class to include appropriate jQuery code and
styles when a sortable table is requested. The head
, body
, and foot
containers
maintain the order items are added, other containers such as styles, CSS fles, javascript,
javascript files are check for duplicates but do not in general maintain the order in
which they were added.
The PageItem
class is an abstract base class for all items added to the body and footer
sections. Children are loosely divided into PageItems
and FormItems
where the
FormItems
are used to pass input fields.
The Form
class is a container for HTML forms, it may contain both PageItems
and
FormItems
.
Page class top container for HTML
PageItem - encapsulates non form input tags
- class ja_webutils.PageItem.PageItem(name='unknown', id='', class_name=None, title=None)
Base class for html tags
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- abstract get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemArray(use_div=False, **kwargs)
A list of PageItems that can be used anywhere a single Item can. Array so it is not confused with PageItemList which is an HTML ordered or unordered list
- add(thing)
add an item to the list
- Parameters:
thing – A PageItem subclass or anything that can be converted to a string
- Return PageItemList:
this object ref so adds can be chained
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Get html representing every PageItem in the array :return str: the html representation of these items
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
Give each item in this array a chance to add to headers
- Parameters:
page (Page) – the Page object we are a part of
- Returns:
None
- class ja_webutils.PageItem.PageItemBlanks(n=1)
Class representing 1 or more blank lines
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemHeader(text=None, level=1, name=None, **kwargs)
“HTML header is a CSS class for emphasis
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemHorizRule(name='unknown', id='', class_name=None, title=None)
Class representing a hoizontl rule
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemImage(url=None, alt_text=None, width=None, height=None, mime=None, **kwargs)
Class representing an image in the page
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Get the HTML representation
- Returns:
str with html of this image
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemLink(url=None, contents=None, target=None, **kwargs)
Class representing a clickable image as a link
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
get the html for this object :return: str html to add to the page
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemList(**kwargs)
A base class for HTML Ordered [number] and Unordered (bullet) list
- add(thing)
Add an item to the list
- Parameters:
thing – A PageItem object or a Python construct that can be converted to a string
- Return PageItemList:
reference to this object so add calls can be chained
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Generate html for this item
- Return str:
the html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- abstract tag_close()
get list closing tag
- Return str:
closing tag
- abstract tag_open()
Get the tag opening eg: <ol type=I> or <ul style=”list-style-type:circle”;>
- Return str:
opening tag
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemOList(type='1', reversed=False, start=None, **kwargs)
Class representing an HTML unordered (bulleted) list
- add(thing)
Add an item to the list
- Parameters:
thing – A PageItem object or a Python construct that can be converted to a string
- Return PageItemList:
reference to this object so add calls can be chained
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Generate html for this item
- Return str:
the html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- tag_close()
close the tag
- Return str:
closing tag
- tag_open()
Generate opening tag for this list
- Return str:
html opening tag
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemRadioButton(prompt=None, options=None, **kwargs)
Radio buttons select one from a list see: https://www.w3schools.com/tags/att_input_type_radio.asp
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_option(id, label, value)
param str label: text user will see param str value: text returned if selected
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemString(thing=None, escape=True, paragraph=False, **kwargs)
Class representing a string or a paragraph
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemUList(marker='disc', **kwargs)
Class representing an HTML unordered (bulleted) list
- add(thing)
Add an item to the list
- Parameters:
thing – A PageItem object or a Python construct that can be converted to a string
- Return PageItemList:
reference to this object so add calls can be chained
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Generate html for this item
- Return str:
the html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- tag_close()
close the UL tag
- Return str:
closing tag
- tag_open()
Generate opening tag for this list
- Return str:
html opening tag
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageItem.PageItemVideo(src=None, autoplay=None, controls=None, height=None, loop=None, muted=None, poster=None, preload=None, width=None, **kwargs)
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
PageForm - encapsulates input tags
Packages for html forms and input fields
A class representing the HTML form it is a container for elements of the form which are defined in their own classes. Other items such as tables, labels, images or links can be added.
The basic parts to a form are:
Head - defines the form and submit action
Hidden - hidden variables are passed back as is but not displayed to the user in any way
Display - pageItems some may be entry some tables or text or images with no returned data
End - Adds submit and cancel buttons (maybe) and closes the form tag
The head section Defines the encoding and the submitted action
You can add as many hidden variables as you wish. They are returned with the form but the user is not shown these variables. Note that they are easily seen and modified by a motivated user so they should not contain anything secret.
The display section may contain any PageItem you wish. It is common to arrange the input items in the table with label column, input item, helpful comment on each row.
- class ja_webutils.PageForm.PageForm(action=None, method='post', nosubmit=False, **kwargs)
nosubmit - True means do not add a sbmit button
- add(it)
Add any acceptable PageItem or python basic data type to form
- Param:
it - any PageItem capable thing
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
Hidden values are sent with the forms but not displayed :param str name: parameter name can not be None :param str value: string value to return or None :return: None
- add_line(it, n=1)
Aa the item and a line break as a convenience :param int n: number of blank lines :param it: any PageItem capable thing :return:
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_form_end(bldr)
Close the form definition :param StringBuilder bldr: where to add html :return: None but bldr is updated
Add hidden variables :param StringBuilder bldr: where to add html :return: None but bldr is updated
- get_form_start(bldr)
Get the opening html for the form :param StringBuilder bldr: buffer to wich to add our html :return: None but bldr is updated
- get_html()
Produce the html for the form :return str: html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
Some items in our list may need to add javascript, or css to the Page :param Page page: paage we are part of :return: None
- class ja_webutils.PageForm.PageFormButton(name=None, contents='', value='', type='submit', enabled=True, target=None, **kwargs)
Represens a button element, depending on type it may be similar to the input type submit but is more flexible in content
Attributes:
text - what is shown in the buttonvalue - what is returned on submiticon - PageItemImage used as the button’s iconenabled - buttons can be disabledtarget - where to display response _self is default, _blank for new tab/window type - button type can be submit, button, reset
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- set_type(new_type)
Set tye button type attri ute with validity check :param str new_type: new button type maybe submit, button, or reset :return: None
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormCheckBox(txt='', checked=False, **kwargs)
A boolean object that appears as a check box
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Get html for this tag :return str: html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormFileUpload(name='', allow_multiple=False, accept=None)
Sets up the upload
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Get the tag :return str: the html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormItem(prompt=None, **kwargs)
prompt - convenience to put string before actual item
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormRadio(prompt=None, **kwargs)
Radio Button class Attributes:
options - list of tuples defining the radio optionsmulti_allowed - allow more than one selection
- add(name=None, value=None, selected=False)
Add an option to the radio group :param str name: option name :param str value: value returned if selected :param boolean selected: initial selection state :return: None
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Return the html tags
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormSelect(name=None, opts=None, mult_allowed=False, **kwargs)
Represents an HTML drop down menu Attributes:
options - list of tuples of name, value, selectedmult_allowed - True -> user may select more than one option
- add(opts)
Add one or more options to the menu
- Parameters:
opts (multiple) – string or list of strings that make up the menu
- Returns:
None
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
return html for ths menu :return str:
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormSubmit(name, value)
Represents the html input submit tag. A button to submit or cancel a form
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Get the html tag for a stadard submit button :return str: html
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
This method is called before adding its html. If the item needs a javascript or css file to be added to the header it can be done here
- Param:
page, the relevant Page object
- Raises:
WebUtilError
- class ja_webutils.PageForm.PageFormText(name=None, default_value='', place_holder='', maxlen=0, size=0, **kwargs)
Text input maxleen: limit on how mlarge of a string may be entereddefault_value - prefilled textpassword - obfuscate entry if truenlines - how many lines to prsentplace_holder - text that does not return, like ‘put uour name here’use_editor - enable jquery’s text editor
- add_classname(cname)
Add a class to the tag attributes for CSS
- Parameters:
cname (str) – class name
- Returns:
None
- add_event(evt_name, call)
Add a call to a javascript routine on a specific event like click
- Parameters:
evt_name (str) – name of event see list in __init__
call (str) – name of jacascript function to call
- Returns:
None
- Raises:
WebUtilError if event is not recognized
- add_style(style, val)
Add a CSS like styles to this tag :param str style: name of styles as defined in the page’s styles sheet :param str val: value for the setting :returns: None :raises: WebUtilError if styles is already set
- static escape(instr)
Escape a string for html
- Parameters:
instr (str) – string to escape
- Returns str:
escaped string
- get_attributes()
Generat the common attributes fot this tag
- Returns str:
common attributes
- get_html()
Each item must define this method to return a complete html tag
- Returns:
simple string with the html tag
- Raises:
WebUtilError
- static get_page_item(thing)
Many methods need a PageItem subclass. This method will verify the argument is a PageItem if not it creates and returns a PageItemString
- Parameters:
thing – aPageItem or any Python construct that can be converted to a string
- Returns:
a PageItem representng the thing
- Raises:
WebUtilError if string conversion fails
- update_header(page)
If etidable add js editor to header :param Page page: :return: